unity实战之linerrender自定义绘画
unity实战之使用LineRenderer自定义画线
由于画板ui是在Canvas层,而LineRenderer画线是在世界空间中。如果按照层级显示,先渲染场景LineRenderer层,再渲染UI层,会导致画线被ui挡住。
为了让LineRenderer显示在UI层上方,我们考虑使用双摄像机来处理。简而言之就是为LineRenderer单独安排一个摄像机用于专门去渲染Line Renderer。
ui
1.创建Camera,命名为LineCamera,调整参数如下:
Clear Flags:Depth only
Projection:Orthographic
其他参数根据实际情况调整。
2.创建Line Renderer,命名为Liner。
3.新建一个多边形碰撞器Ploygon Collider 2D,命名为bg,调节碰撞区域。并为其添加Line Renderer Ctrl脚本,并为其MapCamera,go赋值如下:
MapCamera:LineCamera
go:Liner
4.创建绘画拖尾效果,使用拖尾特效UI_tuowei_10,挂载在bg下。
代码
local view = baseview:new("test2")
local base = view
local ui = nil
function view:start()
model = base.model
ui = base.group
ui = {}
ui.camera = utils.loadinstobj("hero","LineCamera",Layer.MapRoot)
ui.camera.transform.position = Vector3.zero
ui.Liner = utils.findobj(ui.camera,"Liner")
ui.bg = utils.findobj(ui.camera,"bg")
ui.line_ctrl = ui.bg:GetComponent("LineRendererCtrl")
ui.tuowei = utils.findobj(ui.camera,"bg/UI_tuowei_10")
ui.line_ctrl.onComplet = base.on_draw_complet
ui.line_ctrl.onDrag = base.draw_onDrag
end
function base:commit()
ui.Liner:SetActive(true)
ui.line_ctrl:Ready()
base.RefreshUI()
end
function base.RefreshUI()
end
function base.draw_onDrag(go,v3)
--绘画拖尾效果
ui.tuowei:SetActive(true)
ui.tuowei.transform.localPosition = v3
end
function base.on_draw_complet()
ui.tuowei:SetActive(false)
ui.line_ctrl:ScaleDrawing(0,0.25)
-- --画完之后隐藏绘画
-- ui.Liner:SetActive(false)
ui.line_ctrl:Ready()
end
function view:destroy()
ui = nil
renders = {}
build_list = {}
build_data = nil
end
return view
效果预览
LineRendererCtrl脚本
using DG.Tweening;
using LuaInterface;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class LineRendererCtrl : MonoBehaviour,IPointerDownHandler, IPointerUpHandler, IBeginDragHandler, IDragHandler, IEndDragHandler
{
public LuaFunction onComplet;
public LuaFunction onDrag;
public Camera MapCamera;
public GameObject go;
public bool isstart = false;
public bool isclose = true;
// public float scale = 0.5f;
public float pos_z = 100;
private LineRenderer line;
public Vector3[] points;
private float time = 0;
public float max_time = 30;
List<Vector3> pos = new List<Vector3>();
void Awake()
{
points = pos.ToArray();
line = go.GetComponent<LineRenderer>();
// line.startColor = Color.blue;
// line.endColor = Color.blue;
// line.startWidth = 0.1f;
// line.endWidth = 0.1f;
}
public void SetColor(Color c1,Color c2)
{
if (line == null) return;
line.startColor = c1;
line.endColor = c2;
}
public void SetWidth(float f1, float f2)
{
if (line == null) return;
line.startWidth = f1;
line.endWidth = f2;
}
public void Clear()
{
pos.Clear();
points = pos.ToArray();
}
public void Ready()
{
isclose = false;
time = 0;
Clear();
line.positionCount = points.Length;
line.SetPositions(points);
}
void OnDestroy()
{
Clear();
if (onComplet != null)
{
onComplet.Dispose();
onComplet = null;
}
if (onDrag != null)
{
onDrag.Dispose();
onDrag = null;
}
isstart = false;
isclose = true;
}
void Update()
{
if (isclose) return;
if (!isstart) return;
time += Time.deltaTime;
// Util.Log("time+++" + time);
if (time >= max_time)
{
isstart = false;
time = 0f;
EndDrawing();
return;
}
//Util.Log("GetMouseButton+++" + Input.GetMouseButton(0).ToString());
//if (Input.GetMouseButton(0))
//{
// Vector3 result = MapCamera.ScreenToWorldPoint(Input.mousePosition);
// Util.Log("GetMouseButtonDown+++" + time);
// pos.Add(result);
// points = pos.ToArray();
//}
Drawing();
}
public void ScaleDrawing(float scale,float _time)
{
if (line != null && points.Length != 0)
{
Tween _tween = DOTween.To(() => 1, _v => ToScale(_v), scale, _time);
_tween = _tween.SetEase(Ease.InQuart);
_tween.PlayForward();
}
}
public void ToScale(float scale)
{
if (line != null && points.Length != 0)
{
for (int i = 0; i < points.Length; i++)
{
points[i] = new Vector3(points[i].x*scale, points[i].y*scale, points[i].z);
}
line.positionCount = points.Length;
line.SetPositions(points);
}
}
public void EndDrawing()
{
if (isclose) return;
isclose = true;
if (line != null && points.Length != 0)
{
// for (int i = 0; i < points.Length; i++)
// {
// points[i] = new Vector3(points[i].x*scale, points[i].y*scale, points[i].z);
// }
line.positionCount = points.Length;
line.SetPositions(points);
}
if (onComplet != null)
onComplet.Call(points);
}
public void Drawing()
{
if (line!=null && points.Length != 0)
{
// Util.Log("Length+++" + points.Length);
//line.SetVertexCount(points.Length);
line.positionCount = points.Length;
line.SetPositions(points);
}
}
public void OnBeginDrag(PointerEventData eventData)
{
if (isclose) return;
// Util.Log("LineRendererCtrl OnBeginDrag");
isstart = true;
}
public void OnDrag(PointerEventData eventData)
{
// Util.Log("LineRendererCtrl OnDrag");
if (isclose) return;
if (!isstart) return;
//Util.Log(eventData.pointerEnter.name);
if (eventData.pointerEnter==null) return;
if (eventData.pointerEnter.name!="bg")
{
isstart = false;
EndDrawing();
return;
}
Vector3 result = MapCamera.ScreenToWorldPoint(eventData.position);
if (onDrag != null)
onDrag.Call(gameObject, result);
result.z = pos_z;
pos.Add(result);
points = pos.ToArray();
}
public void OnEndDrag(PointerEventData eventData)
{
isstart = false;
Util.Log("LineRendererCtrl OnEndDrag");
//EndDrawing();
}
public void OnPointerDown(PointerEventData eventData)
{
if (isclose) return;
Util.Log("LineRendererCtrl OnPointerDown");
isstart = true;
}
public void OnPointerUp(PointerEventData eventData)
{
Util.Log("LineRendererCtrl OnPointerUp");
isstart = false;
EndDrawing();
}
}
标题:unity实战之linerrender自定义绘画
作者:shirlnGame
地址:https://www.mmzsblog.cn/articles/2024/03/26/1711436649205.html
如未加特殊说明,文章均为原创,转载必须注明出处。均采用CC BY-SA 4.0 协议!
本网站发布的内容(图片、视频和文字)以原创、转载和分享网络内容为主,如果涉及侵权请尽快告知,我们将会在第一时间删除。若本站转载文章遗漏了原文链接,请及时告知,我们将做删除处理!文章观点不代表本网站立场,如需处理请联系首页客服。• 网站转载须在文章起始位置标注作者及原文连接,否则保留追究法律责任的权利。
• 公众号转载请联系网站首页的微信号申请白名单!
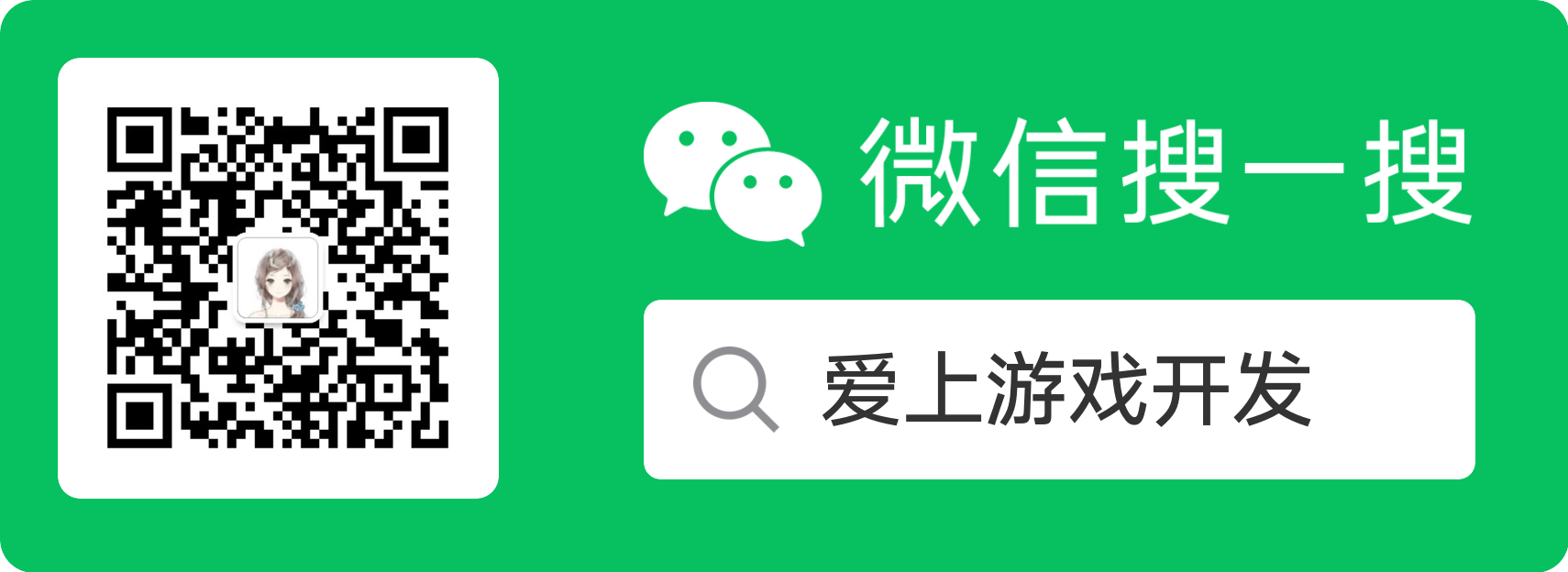