Unity游戏开发工具函数(一)
今天来给大家推荐一些开发过程中经常会用到的一些工具函数,希望对你们能有所帮助!
系统工具
获取本机Mac地址
// 获取本机Mac地址
public static string GetMacAddress()
{
try
{
NetworkInterface[] nis = NetworkInterface.GetAllNetworkInterfaces();
for (int i = 0; i < nis.Length; i++)
{
if (nis[i].Name == "本地连接")
{
return nis[i].GetPhysicalAddress().ToString();
}
}
return "null";
}
catch
{
return "null";
}
}
获取本机Ip地址
//获取本机Ip地址
public static string GetLocalIP()
{
//Dns.GetHostName()获取本机名Dns.GetHostAddresses()根据本机名获取ip地址组
IPAddress[] ips = Dns.GetHostAddresses(Dns.GetHostName());
foreach (IPAddress ip in ips)
{
if (ip.AddressFamily == AddressFamily.InterNetwork)
{
return ip.ToString(); //ipv4
}
}
return null;
}
MD5 32位加密
// MD5 32位加密
public static string UserMd5(string str)
{
string cl = str;
StringBuilder pwd = new StringBuilder();
MD5 md5 = MD5.Create();//实例化一个md5对像
// 加密后是一个字节类型的数组,这里要注意编码UTF8/Unicode等的选择
byte[] s = md5.ComputeHash(Encoding.UTF8.GetBytes(cl));
// 通过使用循环,将字节类型的数组转换为字符串,此字符串是常规字符格式化所得
for (int i = 0; i < s.Length; i++)
{
// 将得到的字符串使用十六进制类型格式。格式后的字符是小写的字母,如果使用大写(X)则格式后的字符是大写字符
pwd.Append(s[i].ToString("x2"));
//pwd = pwd + s[i].ToString("X");
}
return pwd.ToString();
}
计算字符串的MD5值
// 计算字符串的MD5值
public static string md5(string source)
{
MD5CryptoServiceProvider md5 = new MD5CryptoServiceProvider();
byte[] data = System.Text.Encoding.UTF8.GetBytes(source);
byte[] md5Data = md5.ComputeHash(data, 0, data.Length);
md5.Clear();
string destString = "";
for (int i = 0; i < md5Data.Length; i++)
{
destString += System.Convert.ToString(md5Data[i], 16).PadLeft(2, '0');
}
destString = destString.PadLeft(32, '0');
return destString;
}
public static string md5file(string file)
{
try
{
FileStream fs = new FileStream(file, FileMode.Open);
string size = fs.Length / 1024 + "";
Debug.Log("当前文件的大小: " + file + "===>" + (fs.Length / 1024) + "KB");
System.Security.Cryptography.MD5 md5 = new System.Security.Cryptography.MD5CryptoServiceProvider();
byte[] retVal = md5.ComputeHash(fs);
fs.Close();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < retVal.Length; i++)
{
sb.Append(retVal[i].ToString("x2"));
}
return sb + "|" + size;
}
catch (Exception ex)
{
throw new Exception("md5file() fail, error:" + ex.Message);
}
}
字节加密/解密 异或处理
// 字节加密/解密 异或处理
public static void Encypt(ref byte[] targetData, byte m_key)
{
//加密,与key异或,解密的时候同样如此
int dataLength = targetData.Length;
for (int i = 0; i < dataLength; ++i)
{
targetData[i] = (byte)(targetData[i] ^ m_key);
}
}
获取系统时间
//获取系统时间
public static long GetNowTime()
{
TimeSpan ts = new TimeSpan(DateTime.UtcNow.Ticks - new DateTime(1970, 1, 1, 0, 0, 0).Ticks);
return (long)ts.TotalMilliseconds;
}
Base64编码
// Base64编码
public static string Encode(string message)
{
byte[] bytes = Encoding.GetEncoding("utf-8").GetBytes(message);
return Convert.ToBase64String(bytes);
}
Base64解码
// Base64解码
public static string Decode(string message)
{
byte[] bytes = Convert.FromBase64String(message);
return Encoding.GetEncoding("utf-8").GetString(bytes);
}
生成随机字符串
//生成随机字符串
public static string RandomCharacters()
{
string str = string.Empty;
long num2 = DateTime.Now.Ticks + 0;
System.Random random = new System.Random(((int)(((ulong)num2) & 0xffffffffL)) | ((int)(num2 >> 1)));
for (int i = 0; i < 20; i++)
{
char ch;
int num = random.Next();
if ((num % 2) == 0)
{
ch = (char)(0x30 + ((ushort)(num % 10)));
}
else
{
ch = (char)(0x41 + ((ushort)(num % 0x1a)));
}
str = str + ch.ToString();
}
return str;
}
延时执行
延时执行
//延时执行
/// <summary>
/// 延时执行
/// </summary>
/// <param name="action">执行的代码</param>
/// <param name="delaySeconds">延时的秒数</param>
public static Coroutine DelayExecute(this MonoBehaviour behaviour, Action action, float delaySeconds)
{
Coroutine coroutine = behaviour.StartCoroutine(DelayExecute(action, delaySeconds));
return coroutine;
}
private static IEnumerator DelayExecute(Action action, float delaySeconds)
{
yield return YieldInstructioner.GetWaitForSeconds(delaySeconds);
action();
}
等待执行
/// <summary>
/// 等待执行
/// </summary>
/// <param name="action">执行的代码</param>
/// <param name="waitUntil">等待的WaitUntil,返回True</param>
public static Coroutine WaitExecute(this MonoBehaviour behaviour, Action action, WaitUntil waitUntil)
{
Coroutine coroutine = behaviour.StartCoroutine(WaitExecute(action, waitUntil));
return coroutine;
}
private static IEnumerator WaitExecute(Action action, WaitUntil waitUntil)
{
yield return waitUntil;
action();
}
/// <param name="waitUntil">等待的waitWhile,返回false</param>
public static Coroutine WaitExecute(this MonoBehaviour behaviour, Action action, WaitWhile waitWhile)
{
Coroutine coroutine = behaviour.StartCoroutine(WaitExecute(action, waitWhile));
return coroutine;
}
private static IEnumerator WaitExecute(Action action, WaitWhile waitWhile)
{
yield return waitWhile;
action();
}
UI事件/组件
UGUI 控件添加事件监听
/// <summary>
/// UGUI 控件添加事件监听
/// </summary>
/// <param name="target">事件监听目标</param>
/// <param name="type">事件类型</param>
/// <param name="callback">回调函数</param>
public static void AddEventListener(this RectTransform target, EventTriggerType type, UnityAction<BaseEventData> callback)
{
EventTrigger trigger = target.GetComponent<EventTrigger>();
if (trigger == null)
{
trigger = target.gameObject.AddComponent<EventTrigger>();
trigger.triggers = new List<EventTrigger.Entry>();
}
//定义一个事件
EventTrigger.Entry entry = new EventTrigger.Entry();
//设置事件类型
entry.eventID = type;
//设置事件回调函数
entry.callback = new EventTrigger.TriggerEvent();
entry.callback.AddListener(callback);
//添加事件到事件组
trigger.triggers.Add(entry);
}
UGUI Button添加点击事件监听
// UGUI Button添加点击事件监听
public static void AddEventListener(this RectTransform target, UnityAction callback)
{
Button button = target.GetComponent<Button>();
if (button)
{
button.onClick.AddListener(callback);
}
else
{
Debug.Log(target.name + " 丢失了组件 Button!");
}
}
UGUI Button移除所有事件监听
// UGUI Button移除所有事件监听
public static void RemoveAllEventListener(this RectTransform target)
{
Button button = target.GetComponent<Button>();
if (button)
{
button.onClick.RemoveAllListeners();
}
else
{
Debug.Log(target.name + " 丢失了组件 Button!");
}
}
当前鼠标是否停留在UGUI控件上
// 当前鼠标是否停留在UGUI控件上
public static bool IsPointerOverUGUI()
{
if (EventSystem.current)
{
return EventSystem.current.IsPointerOverGameObject();
}
else
{
return false;
}
}
限制Text内容的长度在length以内,超过的部分用replace代替
// 限制Text内容的长度在length以内,超过的部分用replace代替
public static void RestrictLength(this Text tex, int length, string replace)
{
if (tex.text.Length > length)
{
tex.text = tex.text.Substring(0, length) + replace;
}
}
限制Text中指定子字串的字体大小
public static void ToRichSize(this Text tex, string subStr, int size)
{
if (subStr.Length <= 0 || !tex.text.Contains(subStr))
{
return;
}
string valueRich = tex.text;
int index = valueRich.IndexOf(subStr);
if (index >= 0) valueRich = valueRich.Insert(index, "<size=" + size + ">");
else return;
index = valueRich.IndexOf(subStr) + subStr.Length;
if (index >= 0) valueRich = valueRich.Insert(index, "</size>");
else return;
tex.text = valueRich;
}
限制Text中指定子字串的字体颜色
public static void ToRichColor(this Text tex, string subStr, Color color)
{
if (subStr.Length <= 0 || !tex.text.Contains(subStr))
{
return;
}
string valueRich = tex.text;
int index = valueRich.IndexOf(subStr);
if (index >= 0) valueRich = valueRich.Insert(index, "<color=" + color.ToHexSystemString() + ">");
else return;
index = valueRich.IndexOf(subStr) + subStr.Length;
if (index >= 0) valueRich = valueRich.Insert(index, "</color>");
else return;
tex.text = valueRich;
}
限制Text中的指定子字串的字体加粗
// 限制Text中的指定子字串的字体加粗
public static void ToRichBold(this Text tex, string subStr)
{
if (subStr.Length <= 0 || !tex.text.Contains(subStr))
{
return;
}
string valueRich = tex.text;
int index = valueRich.IndexOf(subStr);
if (index >= 0) valueRich = valueRich.Insert(index, "<b>");
else return;
index = valueRich.IndexOf(subStr) + subStr.Length;
if (index >= 0) valueRich = valueRich.Insert(index, "</b>");
else return;
tex.text = valueRich;
}
限制Text中的指定子字串的字体斜体
// 限制Text中的指定子字串的字体斜体
public static void ToRichItalic(this Text tex, string subStr)
{
if (subStr.Length <= 0 || !tex.text.Contains(subStr))
{
return;
}
string valueRich = tex.text;
int index = valueRich.IndexOf(subStr);
if (index >= 0) valueRich = valueRich.Insert(index, "<i>");
else return;
index = valueRich.IndexOf(subStr) + subStr.Length;
if (index >= 0) valueRich = valueRich.Insert(index, "</i>");
else return;
tex.text = valueRich;
}
清除所有富文本样式
// 清除所有富文本样式
public static void ClearRich(this Text tex)
{
string value = tex.text;
for (int i = 0; i < value.Length; i++)
{
if (value[i] == '<')
{
for (int j = i + 1; j < value.Length; j++)
{
if (value[j] == '>')
{
int count = j - i + 1;
value = value.Remove(i, count);
i -= 1;
break;
}
}
}
}
tex.text = value;
}
获取颜色RGB参数的十六进制字符串
// 获取颜色RGB参数的十六进制字符串
public static string ToHexSystemString(this Color color)
{
return "#" + ((int)(color.r * 255)).ToString("x2") +
((int)(color.g * 255)).ToString("x2") +
((int)(color.b * 255)).ToString("x2") +
((int)(color.a * 255)).ToString("x2");
}
查询添加子物体
查找物体的方法
public static Transform FindTheChild(GameObject goParent, string childName)
{
Transform searchTrans = goParent.transform.Find(childName);
if (searchTrans == null)
{
foreach (Transform trans in goParent.transform)
{
searchTrans = FindTheChild(trans.gameObject, childName);
if (searchTrans != null)
{
return searchTrans;
}
}
}
return searchTrans;
}
获取子物体的脚本
//获取子物体的脚本
public static T GetTheChildComponent<T>(GameObject goParent, string childName) where T : Component
{
Transform searchTrans = FindTheChild(goParent, childName);
if (searchTrans != null)
{
return searchTrans.gameObject.GetComponent<T>();
}
else
{
return null;
}
}
查找兄弟
// 查找兄弟
public static GameObject FindBrother(this GameObject obj, string name)
{
GameObject gObject = null;
if (obj.transform.parent)
{
Transform tf = obj.transform.parent.Find(name);
gObject = tf ? tf.gameObject : null;
}
else
{
GameObject[] rootObjs = UnityEngine.SceneManagement.SceneManager.GetActiveScene().GetRootGameObjects();
foreach (GameObject rootObj in rootObjs)
{
if (rootObj.name == name)
{
gObject = rootObj;
break;
}
}
}
return gObject;
}
通过组件查找场景中所有的物体,包括隐藏和激活的
/// <summary>
/// 通过组件查找场景中所有的物体,包括隐藏和激活的
/// </summary>
/// <typeparam name="T">组件类型</typeparam>
/// <param name="Result">组件列表</param>
public static void FindObjectsOfType<T>(List<T> Result) where T : Component
{
if (Result == null) Result = new List<T>();
else Result.Clear();
List<T> sub = new List<T>();
GameObject[] rootObjs = SceneManager.GetActiveScene().GetRootGameObjects();
foreach (GameObject rootObj in rootObjs)
{
rootObj.transform.GetComponentsInChildren(true, sub);
Result.AddRange(sub);
}
}
通过Tag标签查找场景中指定物
//通过Tag标签查找场景中指定物
public static GameObject FindObjectByTag(string tagName)
{
return GameObject.FindGameObjectWithTag(tagName);
}
添加子物体
//添加子物体
public static void AddChildToParent(Transform parentTrs, Transform childTrs)
{
childTrs.SetParent(parentTrs);
childTrs.localPosition = Vector3.zero;
childTrs.localScale = Vector3.one;
childTrs.localEulerAngles = Vector3.zero;
SetLayer(parentTrs.gameObject.layer, childTrs);
}
设置所有子物体的Layer
//设置所有子物体的Layer
public static void SetLayer(int parentLayer, Transform childTrs)
{
childTrs.gameObject.layer = parentLayer;
for (int i = 0; i < childTrs.childCount; i++)
{
Transform child = childTrs.GetChild(i);
child.gameObject.layer = parentLayer;
SetLayer(parentLayer, child);
}
}
标题:Unity游戏开发工具函数(一)
作者:shirlnGame
地址:https://www.mmzsblog.cn/articles/2020/12/19/1608361112509.html
如未加特殊说明,文章均为原创,转载必须注明出处。均采用CC BY-SA 4.0 协议!
本网站发布的内容(图片、视频和文字)以原创、转载和分享网络内容为主,如果涉及侵权请尽快告知,我们将会在第一时间删除。若本站转载文章遗漏了原文链接,请及时告知,我们将做删除处理!文章观点不代表本网站立场,如需处理请联系首页客服。• 网站转载须在文章起始位置标注作者及原文连接,否则保留追究法律责任的权利。
• 公众号转载请联系网站首页的微信号申请白名单!
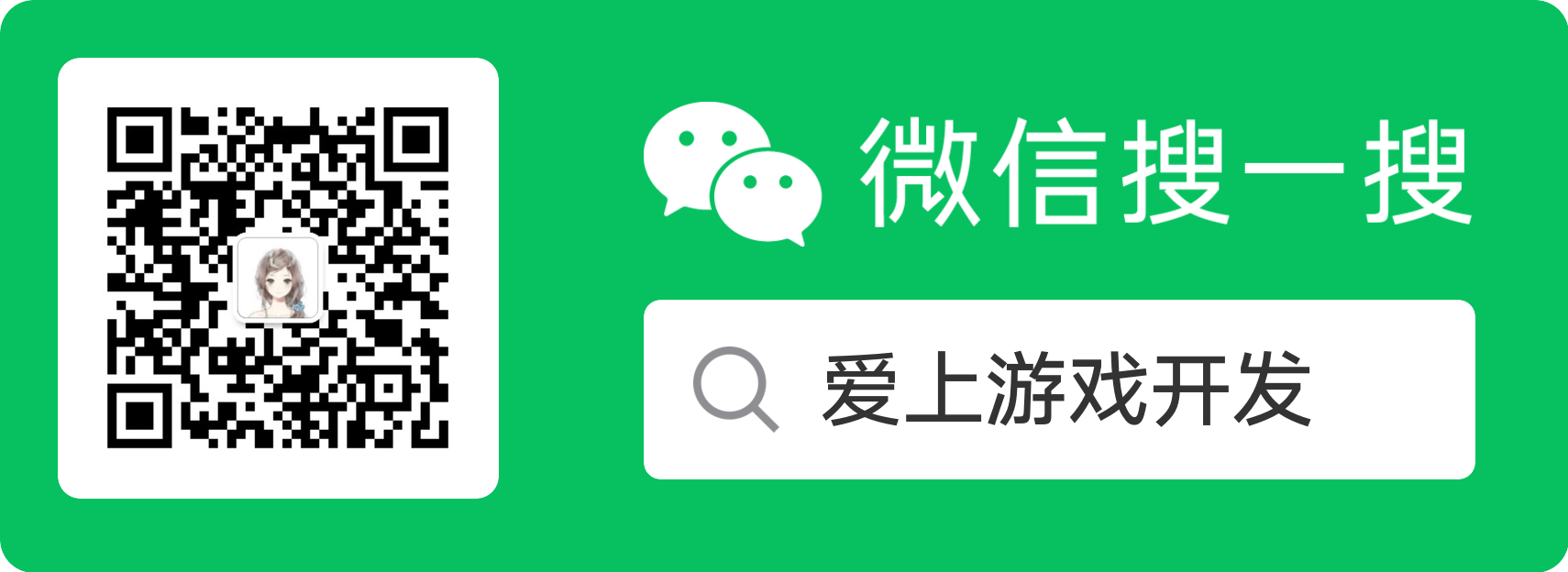