SpringBoot集成mybatis多数据源配置(Druid版)
SpringBoot集成mybatis自定义多数据源配置
1.首先配置application.yml
因为spring官方没有提供和durid集成,所以这部分也需要自定义配置。
datasource:
local:
url: jdbc:mysql://127.0.0.1:3306/my_dev?autoReconnect=true&useUnicode=true&characterEncoding=UTF-8&useSSL=false
driver-class-name: com.mysql.jdbc.Driver
username: root
password: 123456
remote:
url: jdbc:mysql://127.0.0.1:3306/my_dev?autoReconnect=true&useUnicode=true&characterEncoding=UTF-8&useSSL=false
driver-class-name: com.mysql.jdbc.Driver
username: root
password: 123456
druid:
#初始化大小,最小,最大
initial-size: 5
max-active: 10
min-idle: 5
#配置获取连接等待超时的时间
max-wait: 6000
#检测连接是否有效的sql
validation-query: "select '1'"
validation-query-timeout: 2000
test-on-borrow: false
test-on-return: false
test-while-idle: true
#配置间隔多久才进行一次检测,检测需要关闭的空闲连接,单位是毫秒
time-between-eviction-runs-millis: 600000
#配置一个连接在池中最小生存的时间,单位是毫秒
min-evictable-idle-time-millis: 300000
remove-abandoned: true
2.Druid基础配置
用于将yml里的配置映射进来,属性名采用驼峰式。
@Getter
@Setter
@ConfigurationProperties(prefix = "datasource.druid")
public class DruidConfigProperties {
private int initialSize;
private int maxActive;
private int minIdle;
private int maxWait;
private String validationQuery;
private int validationQueryTimeout;
private boolean testOnBorrow;
private boolean testOnReturn;
private boolean testWhileIdle;
private long timeBetweenEvictionRunsMillis;
private long minEvictableIdleTimeMillis;
private boolean removeAbandoned;
}
3.配置DruidDataSource
@Configuration
@EnableConfigurationProperties(DruidConfigProperties.class)
public class DataSourceConfiguration {
private final Environment env;
private final DruidConfigProperties poolProps;
public DataSourceConfiguration(Environment env, DruidConfigProperties poolProps) {
this.env = env;
this.poolProps = poolProps;
}
@Bean
public DataSource localDataSource(){
DruidDataSource dataSource=createDataSource("local");
configPool(dataSource,poolProps);
LOGGER.debug("数据源对象初始化完成->local:{}",dataSource.toString());
return dataSource;
}
@Bean
public DataSource remoteDataSource(){
DruidDataSource dataSource=createDataSource("remote");
configPool(dataSource,poolProps);
LOGGER.debug("数据源对象初始化完成->remote:{}",dataSource.toString());
return dataSource;
}
//加载durid配置
private void configPool(DruidDataSource dataSource,DruidConfigProperties poolProps){
dataSource.setInitialSize(poolProps.getInitialSize());
dataSource.setMaxActive(poolProps.getMaxActive());
dataSource.setMinIdle(poolProps.getMinIdle());
dataSource.setMaxWait(poolProps.getMaxWait());
dataSource.setMinEvictableIdleTimeMillis(poolProps.getMinEvictableIdleTimeMillis());
dataSource.setValidationQuery(poolProps.getValidationQuery());
dataSource.setValidationQueryTimeout(poolProps.getValidationQueryTimeout());
dataSource.setTestWhileIdle(poolProps.isTestWhileIdle());
dataSource.setRemoveAbandoned(poolProps.isRemoveAbandoned());
dataSource.setTestOnBorrow(poolProps.isTestOnBorrow());
dataSource.setTestOnReturn(poolProps.isTestOnReturn());
}
//根据dataSourceName加载对应的数据库信息
private DruidDataSource createDataSource(String dataSourceName){
String template = "datasource.%s.%s";
DruidDataSource dataSource = new DruidDataSource();
dataSource.setUrl(env.getProperty(String.format(template,dataSourceName,"url")));
dataSource.setUsername(env.getProperty(String.format(template,dataSourceName,"username")));
dataSource.setPassword(env.getProperty(String.format(template,dataSourceName,"password")));
dataSource.setDriverClassName(env.getProperty(String.format(template, dataSourceName, "driver-class-name")));
return dataSource;
}
}
首先根据dataSourceName通过从环境变量中获取数据源的url,username,password和driver,然后在将刚才的Druid基础配置通过@EnableConfigurationProperties(DruidConfigProperties.class)
加载进来,并set到dataSource里,最后返回dataSource。
4.配置mybatis
@Configuration
//该注解的参数对应的类必须存在,否则不解析该注解修饰的配置类
@ConditionalOnClass({ SqlSessionFactory.class, SqlSessionFactoryBean.class })
public class MybatisConfiguration {
//第一个数据源
@Configuration
@MapperScan(basePackages = {"com.mmzsblog.learn.mybatis.mapper.local"},
sqlSessionFactoryRef = "localSqlSessionFactory")
public static class LocalMybatisConfig{
private final DataSource dataSource;
public LocalMybatisConfig(@Qualifier("localDataSource") DataSource dataSource) {
this.dataSource = dataSource;
}
//创建SqlSessionFactory
@Bean
public SqlSessionFactory localSqlSessionFactory() throws Exception {
SqlSessionFactoryBean factoryBean = new SqlSessionFactoryBean();
factoryBean.setDataSource(dataSource);
factoryBean.setMapperLocations(new PathMatchingResourcePatternResolver()
.getResources("classpath:/mapper/local/*.xml"));
//factoryBean.setConfigLocation(new ClassPathResource("/mapper/mybatis-config.xml"));
return factoryBean.getObject();
}
//利用SqlSessionFactory创建SqlSessionTemplate
@Bean
public SqlSessionTemplate localSqlSessionTemplate() throws Exception {
return new SqlSessionTemplate(localSqlSessionFactory());
}
//事务
@Bean
public PlatformTransactionManager localTransactionManager(){
return new DataSourceTransactionManager(dataSource);
}
}
//第二个数据源
@Configuration
@MapperScan(basePackages = {"com.mmzsblog.learn.mybatis.mapper.remote"},
sqlSessionFactoryRef = "remoteSqlSessionFactory")
public static class RemoteMybatisConfig{
private final DataSource dataSource;
public RemoteMybatisConfig(@Qualifier("remoteDataSource") DataSource dataSource) {
this.dataSource = dataSource;
}
//创建SqlSessionFactory
@Bean
public SqlSessionFactory remoteSqlSessionFactory() throws Exception {
SqlSessionFactoryBean factoryBean = new SqlSessionFactoryBean();
factoryBean.setDataSource(dataSource);
factoryBean.setMapperLocations(new PathMatchingResourcePatternResolver()
.getResources("classpath:/mapper/remote/*.xml"));
//factoryBean.setConfigLocation(new ClassPathResource("/mapper/mybatis-config.xml"));
return factoryBean.getObject();
}
//利用SqlSessionFactory创建SqlSessionTemplate
@Bean
public SqlSessionTemplate localSqlSessionTemplate() throws Exception {
return new SqlSessionTemplate(remoteSqlSessionFactory());
}
//事务
@Bean
public PlatformTransactionManager localTransactionManager(){
return new DataSourceTransactionManager(dataSource);
}
}
}
mybatis多数据源配置使用了静态内部类的方式,之后的SqlSession和事务配置和官方提供的没有区别。
其它方式
标题:SpringBoot集成mybatis多数据源配置(Druid版)
作者:mmzsblog
地址:https://www.mmzsblog.cn/articles/2023/06/05/1685967066886.html
如未加特殊说明,文章均为原创,转载必须注明出处。均采用CC BY-SA 4.0 协议!
本网站发布的内容(图片、视频和文字)以原创、转载和分享网络内容为主,如果涉及侵权请尽快告知,我们将会在第一时间删除。若本站转载文章遗漏了原文链接,请及时告知,我们将做删除处理!文章观点不代表本网站立场,如需处理请联系首页客服。• 网站转载须在文章起始位置标注作者及原文连接,否则保留追究法律责任的权利。
• 公众号转载请联系网站首页的微信号申请白名单!
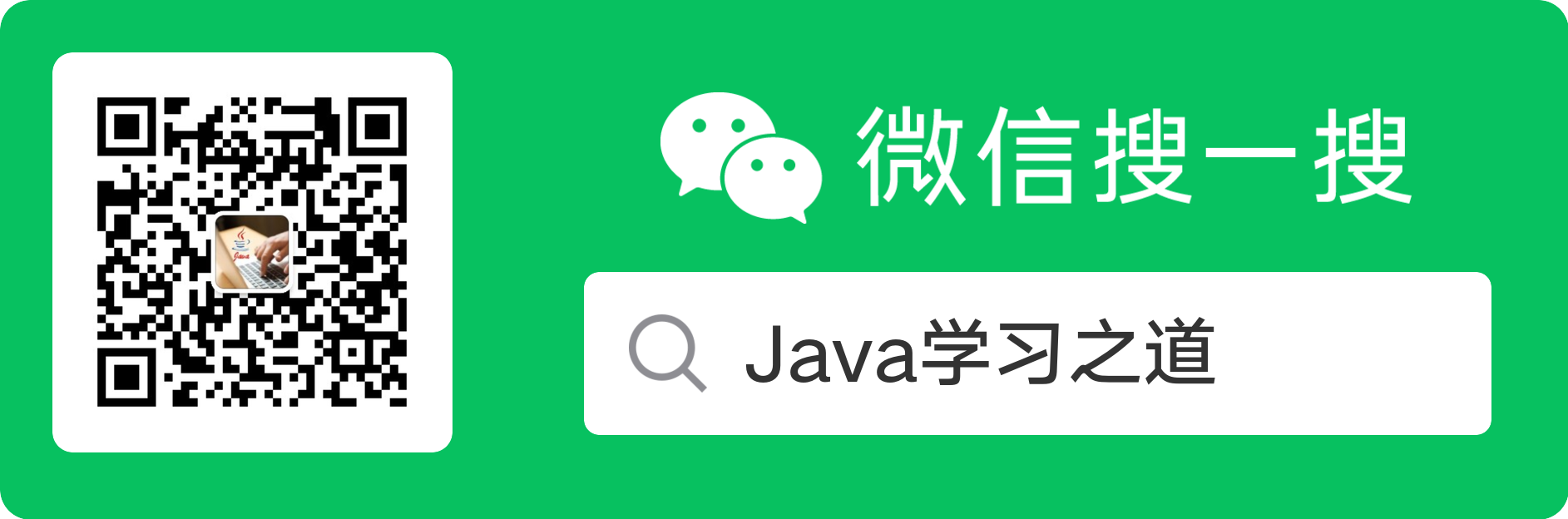